Data structures
Data stuctures
-
Theory
-
Practical work 1
-
Practical work 2
-
Exam style questions
There are several common data structures used in programming languages, and here are some of the most fundamental ones:
1. Arrays: An array is a collection of elements of the same type that are stored in contiguous memory locations. Arrays are used to store data of fixed size, and accessing a specific element in an array is done using its index.
# Define an array of integers
arr = [1, 2, 3, 4, 5]
# Access the third element of the array
print(arr[2])
# Iterate over all elements in the array
for element in arr:
print(element)
Array Data Structure
Example: Arrays are used in many real-life applications, such as in spreadsheet software to store data in rows and columns, in the image and audio processing software to store pixel and sample data, and in databases to store and retrieve data.
2. Linked Lists: A linked list is a data structure consisting of a sequence of nodes, where each node stores a value and a reference to the next node in the list. Linked lists are used to store data of varying sizes, and they provide efficient insertion and deletion operations.
Example: Linked lists are used in many applications where data is stored and accessed sequentially, such as in browser history and navigation, in music and video streaming services to queue and play media, and in operating systems to manage processes and memory.
3. Stacks: A stack is a data structure that stores a collection of elements in a last-in-first-out (LIFO) order. Elements are added and removed from the top of the stack, making it a useful data structure for tasks like parsing expressions and implementing undo/redo functionality.
Example: Stacks are used in many applications where the most recently added element needs to be processed first, such as in web browsers to manage the back and forward buttons, in text editors to implement undo and redo functionality, and in compilers to evaluate expressions.
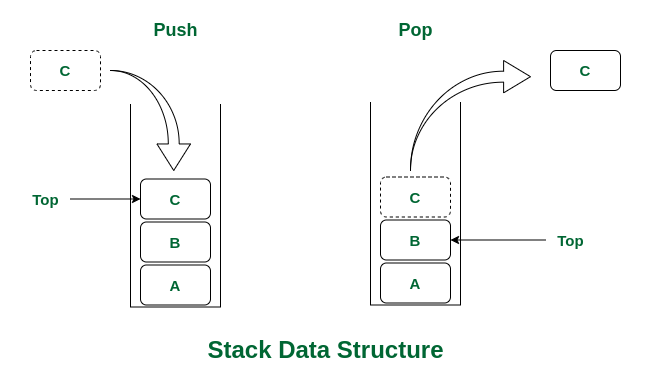
4. Queues: A queue is a data structure that stores a collection of elements in a first-in-first-out (FIFO) order. Elements are added to the back of the queue and removed from the front, making it a useful data structure for implementing systems that process requests in the order they are received.
Example: Queues are used in many applications where data needs to be processed in the order it was received, such as in customer service centers to manage phone and email inquiries, in transportation systems to manage ticket sales and boarding, and in computer networks to manage packet routing.
We define a queue to be a list in which all additions to the list are made at one end, and all deletions from the list are made at the other end. The element which is first pushed into the order, the operation is first performed on that.
5. Trees: A tree is a hierarchical data structure consisting of nodes connected by edges. Each node can have zero or more child nodes, and there is a single node at the top of the hierarchy called the root. Trees are used to represent hierarchical structures like file systems, and they provide efficient search and insertion operations.
Example: Trees are used in many applications where data has a hierarchical structure, such as in file systems to organize files and folders, in HTML and XML documents to represent page layouts and data structures, and in database indexing to optimize queries.
This data structure is a specialized method to organize and store data in the computer to be used more effectively. It consists of a central node, structural nodes, and sub-nodes, which are connected via edges. We can also say that tree data structure has roots, branches, and leaves connected with one another.
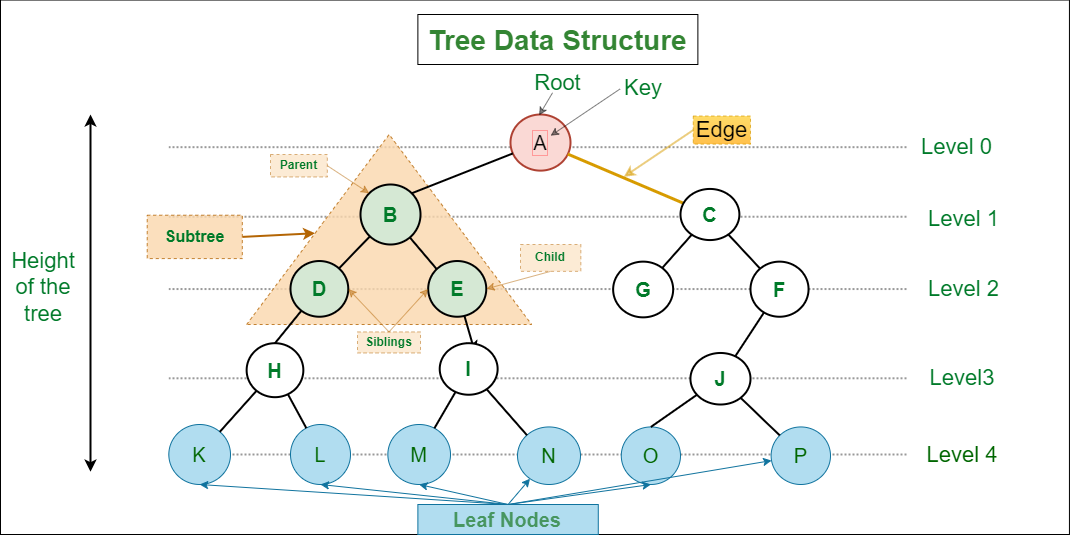
6. Binary tree: Binary Tree is defined as a tree data structure where each node has at most 2 children. Since each element in a binary tree can have only 2 children, we typically name them the left and right child.
Binary Tree Representation
A Binary tree is represented by a pointer to the topmost node (commonly known as the “root”) of the tree. If the tree is empty, then the value of the root is NULL. Each node of a Binary Tree contains the following parts:
- Data
- Pointer to left child
- Pointer to right child
Basic Operation On Binary Tree:
- Inserting an element.
- Removing an element.
- Searching for an element.
- Traversing the tree
- Finding the height of the tree
- Find the level of a node of the tree
- Finding the size of the entire tree.
7. Hash Tables: A hash table is a data structure that stores a collection of key-value pairs. Keys are hashed to determine their index in an array, and values are stored at that index. Hash tables provide efficient lookup and insertion operations, making them a popular choice for implementing dictionaries and caches.
Example: Hash tables are used in many applications where data needs to be stored and accessed quickly and efficiently, such as in search engines to index and retrieve web pages, in social media networks to manage user profiles and content, and in online shopping sites to store and retrieve product information and reviews.
Why use hash tables?
The most valuable aspect of a hash table over other abstract data structures is its speed in performing insertion, deletion, and search operations. Hash tables can do them all in constant time.
For those unfamiliar with time complexity (big O notation), constant time is the fastest possible time complexity. Hash tables can perform nearly all methods (except list) very fast in O(1) time.
Algorithm | Average | Worst case |
---|---|---|
List | O(n) | O(n) |
Search | O(1) | O(n) |
Insert | O(1) | O(n) |
Delete | O(1) | O(n) |
Because of this efficiency, you'll find hash tables to be pretty dang useful for many use cases. And if you look carefully, you’ll notice that they’re actually implemented in a variety of places throughout your tools, like your databases, caches, data-fetching libraries, and so on.
More about hash tables here
Here are some task ideas using one and two-dimensional arrays for beginner, intermediate, and advanced levels:
Level 1:
- Write a program that creates an array of 5 integers and prints out each value in the array.
- Write a program that asks the user for 10 integers, stores them in an array, and then prints out the sum of all the values in the array.
- Write a program that creates a 2D array of size 3x3, initializes each value to 0, and then prints out the array. Find the maximum/minimum/average/second max.
Level 2:
- Write a program that takes in an array of integers and sorts the array in ascending order using the bubble sort/insertion sort algorithm. Find an element using binary search.
- Write a program that creates a 2D array of size 4x4 and then rotates the array 90 degrees clockwise.
- Write a program that takes in two arrays of integers and returns a new array that contains only the elements that appear in both arrays.
1. The table below demonstrates a two-dimensional array that stores 5 shopping carts with 10 product ids each.
15 7 19 3 7 11 17 10 12 16 1 11 3 4 9 18 10 5 9 2 6 19 2 18 16 14 17 3 5 10 4 19 12 2 11 15 6 5 16 1 5 14 15 4 17 3 1 8 16 12
Write code/pseudocode to find the frequency of each product id using a one-dimensional array of size 20.
2. The following data items need to be stored for each student in a group:
• student name (a string)
• test score (an integer).
State a suitable data structure and justify your answer.
Structure ..................................................................................................................................
Justification ..............................................................................................................................
3. A program will:
• input 50 unique integer values
• output the largest value
• output the average of the values excluding the largest value.
Draw a program flowchart to represent the algorithm.
Variable declarations are not required.
It is not necessary to check that each input value is unique.
4. The following diagram represents an Abstract Data Type (ADT) for a linked list.
[ ] ->[ A [ ]->[ C [ ]->[ D [ ] -> [ E [ Ø ]
The free list is as follows:
[ ] ->[ [ ]->[ [ ]->[ [ ] -> [ [ Ø ]
(a) Explain how a node containing data value B is added to the list in an alphabetic sequence [4]
(b) Describe how the linked list in part (a) may be implemented using variables and arrays [2]
5. A global 1D array, ProdNum, of type INTEGER, contains 5000 elements and is used to store product numbers.
A procedure is needed to sort ProdNum into ascending order using a bubble sort algorithm.
Write program code for the procedure BubbleSort().
Visual Basic and Pascal: You should include the declaration statements for variables.
Python: You should show a comment statement for each variable used with its data type.
Programming language ....................................................................................................................
Program code :